
History
Spring framework was created as an alternative to the the complex J2EE specification and particular the usage of Enterprise Java Beans (EJB) that required fully fledged application server such as IBM WebSphere, WebLogic or JBoss. Although J2EE may fit the requirements for big enterprise level devolvement it was too complex and cumbersome for simple applications.
Please note that J2EE provided a full distributed system solution comprehending many like Enterprise Beans, Remote Method Invocation, Transaction managements, ORM, Servlet containers among others.
Spring came out as a lightweight middleware framework for java, based in simple beans (POJO) and relying heavily in the concept of Dependency Injection (DI), the implementation of an Application context that would manage the beans and thus enabling for Inversion of Control (IoC). And also very important Spring applications would run in as simple Servlet container like Tomcat.
At the time there already frameworks and technologies that compete with J2EE in regards to web development (Apache Struts), WebServices (Axis), ORM (Hibernate) to say a few, but the success of Spring came by positioning itself as the framework that couple different technologies and even provider solutions of its own as Spring MVC and JDBC Template.
The join venture of Spring MVC + Spring Core + Hibernate was a very popular solution to a three layer architecture and boosted the popularity of the framework.

https://www.techopedia.com/2/32100/software/a-detailed-look-at-3-tier-software-architecture
In the following years Spring added more functionality and integration capabilities and delivers independent modules that can be added to extend functionality, some of the most popular ones up to date are:
- Spring MVC
- Spring Data JPA
- Spring Security
Curiously, EJB 3.0 specification end up coping much of the ideas brought by Spring but in a standardized fashion.
Configuration
In the earlier years Spring relied in XML configuration, as the later was very popular at the time, with improvements in Java, Spring moved to a Java configuration using @Configuration and @Bean definitions.
The XML configuration was essential for the configuration of containers, Tomcat and others used an web.xml file for servlet configuration and Spring followed in having it’s bean configuration in such format.
There are still some examples of this type of configuration out there:
https://github.com/ajcm/spring-examples/tree/main/examples/spring4-mvc-example
With the introduction of Java configuration the container itself started to be configured as an class (AbstractSecurityWebApplicationInitializer), example:
https://github.com/spring-petclinic/spring-framework-petclinic (without web.xml)
https://github.com/ajcm/spring-examples/tree/main/examples/spring6-hello-mvc-security
In recent years Spring moved to Component and stereotypes annotation and autoscan leaving Java configuration for third party integration or legacy application.
Additionally the tradition MVC workflow was replaced with API and service architecture more focused in microservices and external frontends.
As microservices became more popular, Spring offered a comprehensive set of technologies to address the challenges brought by this type of approach. Thus Spring Netflix/Spring cloud was delivered.
Architecture
https://www.amitph.com/spring-framework-architecture
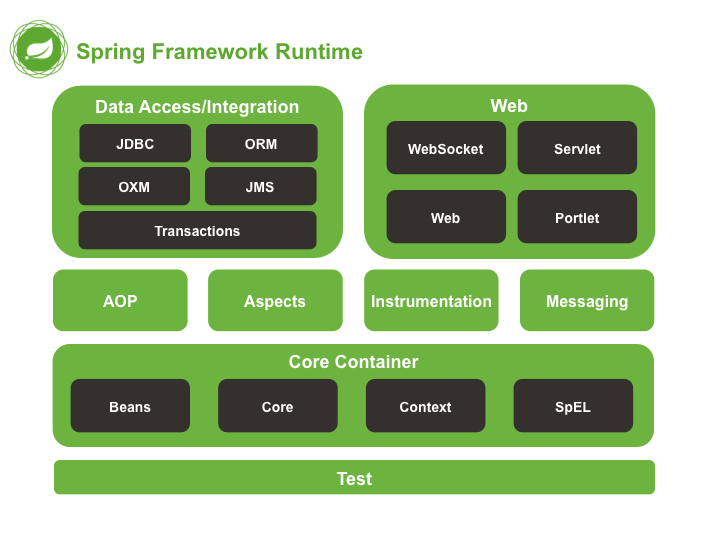
Spring Core
The Core module contains basic Spring Framework classes including Dependency Injection (DI) and Inversion of Control (IOC). The Spring Core is available at Spring Core Repo. No matter which type of Spring Application you are building, you will always have direct or indirect dependency over Spring Core.
Spring Bean
Spring Bean module manages the lifecycle of beans. In the Spring Framework a Bean is any Java Class which is registered with Spring and Spring manages these bean classes. The Spring Bean module has a Bean Factory which creates bean instances, resolves bean to bean dependencies, and auto-wires the beans based on the name, or type.
Spring Bean module can be found on the Spring Beans Repo.
Spring Context
We learnt that Spring Bean are responsible for managing the Spring Beans. These Spring Beans are defined in the context called as a Context. In Spring every objet is a Bean, let it be a config entry or a user defined class (For example Employee). All such beans, their constructors or factory methods and dependencies are defined in the Context. The beans are accessed via Context.
Most of the time the Spring Context is started when a Spring Application starts and hence called as Application Context. Link to Spring Context Repo.
SpEL
The SpEL stands for Spring Expression Language, it a power full expression language. It is used to resolve expressions to values at runtime. SpEL can query objects graphs on runtime and can be used in XML or annotation based Bean Definition and Bean Configuration. The word runtime is really important here, as the expressions can be evaluated based on runtime configuration or values of other expressions.
Can be found at Spring Expression Language Repo.
Spring Web
As it is quite obvious from the name it self, the Spring Web components are used to build web applications. Using the Spring Web module we can build complete MVC applications, interceptors, Web Services, Portlets.