Implementations
Reactive programming is a programming paradigm associated with non-blocking, asynchronous and event driven processing of data streams. It facilitates automatic propagation of data flow.
Implementations: Project Reactor, RxJava, Java9 Reactive Flow.
There are four interfaces:
Publisher
---
subscribe(Subscriver<> subscriber)
Subscriber
---
onSubscrive(Subscription subscription)
onNext(T item)
onError(Throwable t)
onComplete()
Subscription
---
request(Long n)
cancel()
Processor
---
extends Subscriver<T>, Publisher<T>
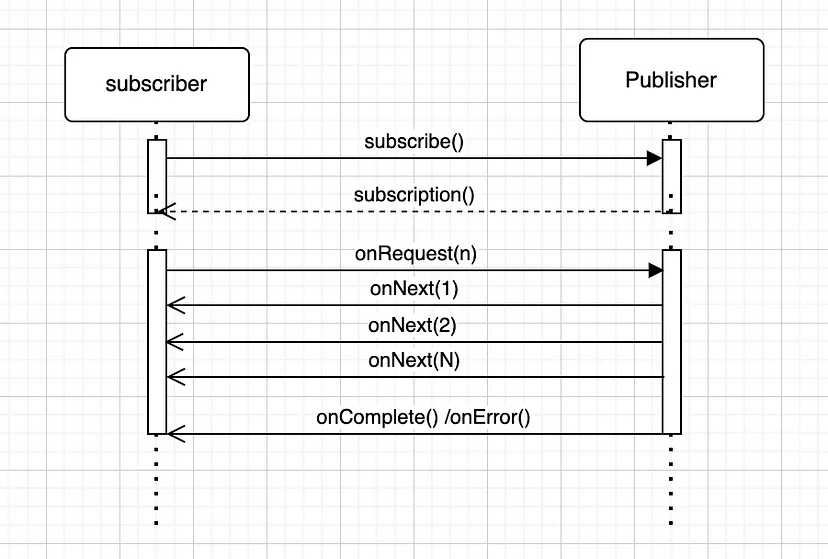
Project Reactor
Spring WebFlux, provides two data types:
- Flux – Publisher 0-N elements
- Mono Publisher 0-1 elements
RxJava
Java extension of ReactiveX
Observables – any object can get data from data source and which state maybe of interest in a way that others can register interest (Publisher).
Observer – any object that wishes to be notified when a state of another object changes.
RxJava Observables
- Observable: 0-N items, not backpressure enabled.
- Flowable: 0-N items, with backpressure.
- Completable: doesn’t emit any item.
- Single: emits only one item or error.
- Maybe: emits zero or one item.
Java9 Reactive Flow
Reactive Streams (java.util.concurrent.Flow)
Publisher, Subscriber, Subscription and Processor interfaces.
Backpressure strategies
- Control the producer
- Buffering (accumulate incoming data)
- Drop (sample a percentage of incoming data)
Some stategies:
Pull – consumer controls producer (1:1 request style, Flowables in RxJava).
Push – producer in control, pushed data to consumer when it’s available.