Spring Security’s method authorization support is handy for:
- Extracting fine-grained authorization logic; for example, when the method parameters and return values contribute to the authorization decision.
- Enforcing security at the service layer
- Stylistically favoring annotation-based over
HttpSecurity
-based configuration
And since Method Security is built using Spring AoP.
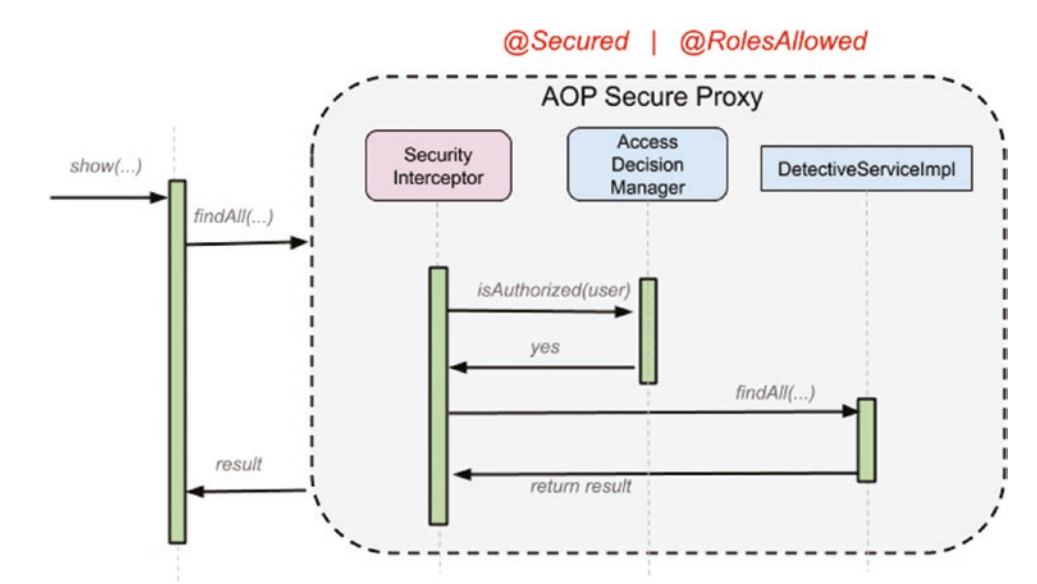
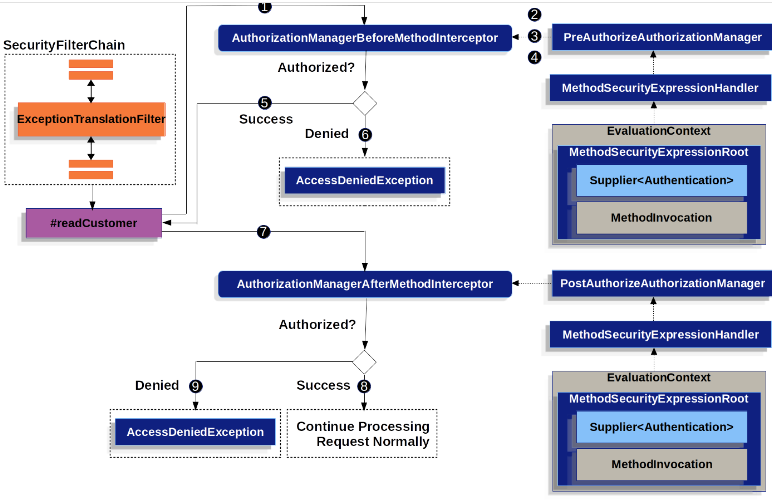
Annotation @EnableMethodSecurity
- prePostEnabled – disabled methods security pre-configuration.
- securedEnabled – enables @Secured (disabled by default)
- jsr250Enabled – enables JSR 250 annotations (disabled by default)
Annotation @Secured is a legacy option to authorize invocations, superseded by @PreAuthorized.
JSR 250 annotations correspond to @RolesAllowed, @PermitAll and @DenyAll.
Annotations
@PreAuthorize and @PostAuthorize – verifies condition before or after method invocation.
@PreFilter and @PostFilter – to filter a collection argument before or after executing the method.
@Secure doesn’t support SpEL
@Secured({ "ROLE_VIEWER", "ROLE_EDITOR" })
public boolean isValidUsername(String username) {
return userRoleRepository.isValidUsername(username);
}
https://www.baeldung.com/spring-security-method-security
Available Expression Fields and Methods
The first thing this provides is an enhanced set of authorization fields and methods to your SpEL expressions. What follows is a quick overview of the most common methods:
permitAll
– The method requires no authorization to be invoked; note that in this case, theAuthentication
is never retrieved from the sessiondenyAll
– The method is not allowed under any circumstances; note that in this case, theAuthentication
is never retrieved from the sessionhasAuthority
– The method requires that theAuthentication
have aGrantedAuthority
that matches the given valuehasRole
– A shortcut forhasAuthority
that prefixesROLE_
or whatever is configured as the default prefixhasAnyAuthority
– The method requires that theAuthentication
have aGrantedAuthority
that matches any of the given valueshasAnyRole
– A shortcut forhasAnyAuthority
that prefixesROLE_
or whatever is configured as the default prefixhasPermission
– A hook into yourPermissionEvaluator
instance for doing object-level authorization
And here is a brief look at the most common fields:
authentication
– TheAuthentication
instance associated with this method invocationprincipal
– TheAuthentication#getPrincipal
associated with this method invocation
Examples
@PreAuthorize("denyAll")
@PreAuthorize("hasRole('ADMIN')")
@PreAuthorize("hasAuthority('db') and hasRole('ADMIN')")
@PreAuthorize("principal.claims['aud'] == 'my-audience'")
@PreAuthorize("@authz.check(authentication, #root)")
@PreAuthorize("hasRole('ROLE_VIEWER') or hasRole('ROLE_EDITOR')")
@PostAuthorize("#username == authentication.principal.username")
@PreFilter("filterObject != authentication.principal.username")
https://docs.spring.io/spring-security/reference/servlet/authorization/method-security.html