Core concepts
Join point – point during the execution of a program, in Spring MVC always represents a method execution.
Pointcut – predicate that matches join points.
Advice – action taken at a particular join point, types of advice include “around”, “after” and “before”.
Aspect – pointcut + advice.
Weaving – linking aspects with other application types or objects to create an advices objects.
Types of advice
Before advice – runs before a join point but doesn’t is able to prevent flow execution (unless throws exception).
AfterReturning – runs after join point completes normally (with no exception).
AfterThrowing – run if method throws exception.
After (finally) – run after either join point returns normally or not.
Around – surrounds a join point.
Supported Pointcut Designators
Spring AOP supports the following AspectJ pointcut designators (PCD) for use in pointcut expressions:
execution
– matches executed method
within – match proxies object type
this – match proxy type
args – match executed method argument types
@target – proxied object type has annotation
@args – method arguments have annotation
@within – proxied object types with annotation
@annotation – execution method has annotation
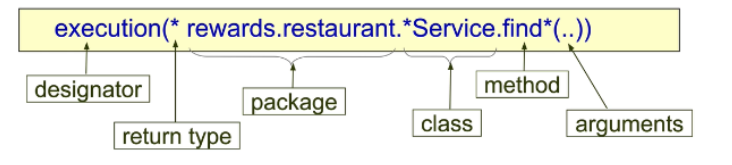
() - matches a method that takes no parameters
(..) - matches any number (zero or more) of parameters.
(*) - pattern matches a method that takes one parameter of any type
(*,String) - matches a method that takes two parameters.
Code example
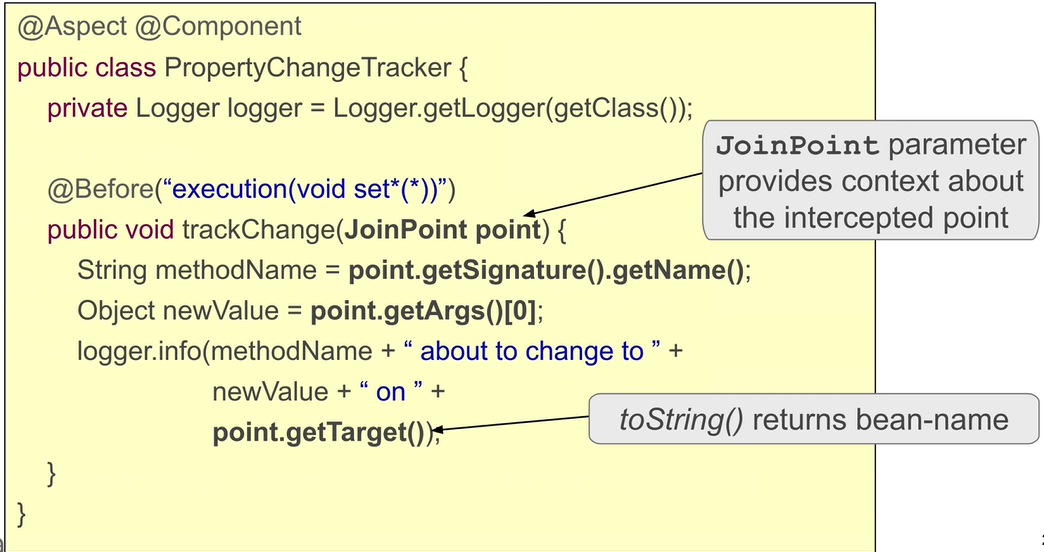
Access to the Current JoinPoint
Any advice method may declare, as its first parameter, a parameter of type
org.aspectj.lang.JoinPoint. Note that around advice is required to declare a first parameter of type
ProceedingJoinPoint, which is a subclass of JoinPoint.
The JoinPoint interface provides a number of useful methods:
- getArgs(): Returns the method arguments.
- getThis(): Returns the proxy object.
- getTarget(): Returns the target object.
- getSignature(): Returns a description of the method that is being advised.
- toString(): Prints a useful description of the method being advised.
ProceedingJoinPoint is a JoinPoint with additional features. ProceedingJoinPoint is used with @Around advice. @Around is very powerful advice that combines the features of rest of the Advice. ProceedingJoinPoint::proceed is basically used to execute the original method.
Proxies
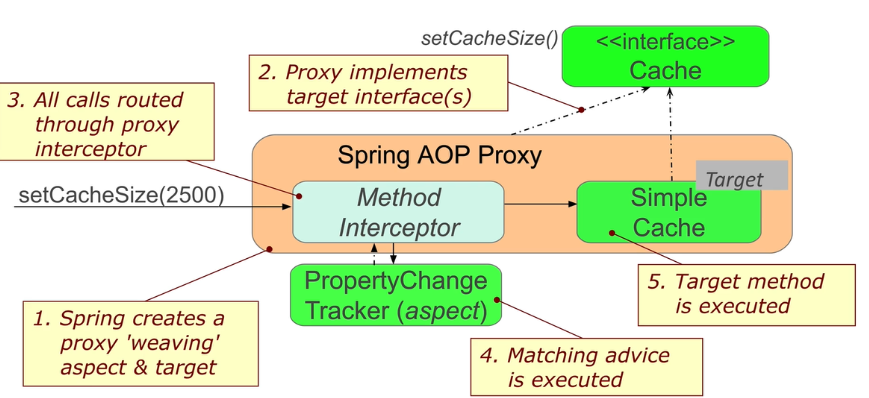
This means that method calls on that object reference are calls on the proxy. As a result, the proxy can delegate to all of the interceptors (advice) that are relevant to that particular method call. However, once the call has finally reached the target object (the SimplePojo reference in this case), any method calls that it may make on itself, such as this.bar() or this.foo(), are going to be invoked against the this reference, and not the proxy. This has important implications. It means that self-invocation is not going to result in the advice associated with a method invocation getting a chance to run.
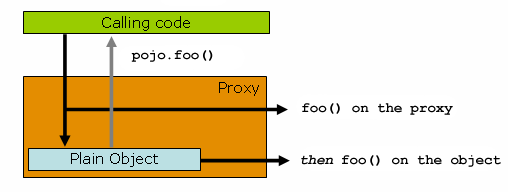
Example
@Aspect
public class ConcurrentOperationExecutor implements Ordered {
private static final int DEFAULT_MAX_RETRIES = 2;
private int maxRetries = DEFAULT_MAX_RETRIES;
private int order = 1;
public void setMaxRetries(int maxRetries) {
this.maxRetries = maxRetries;
}
public int getOrder() {
return this.order;
}
public void setOrder(int order) {
this.order = order;
}
@Around("com.xyz.CommonPointcuts.businessService()")
public Object doConcurrentOperation(ProceedingJoinPoint pjp) throws Throwable {
int numAttempts = 0;
PessimisticLockingFailureException lockFailureException;
do {
numAttempts++;
try {
return pjp.proceed();
}
catch(PessimisticLockingFailureException ex) {
lockFailureException = ex;
}
} while(numAttempts <= this.maxRetries);
throw lockFailureException;
}
}
https://docs.spring.io/spring-framework/reference/core/aop/introduction-defn.html