Concept
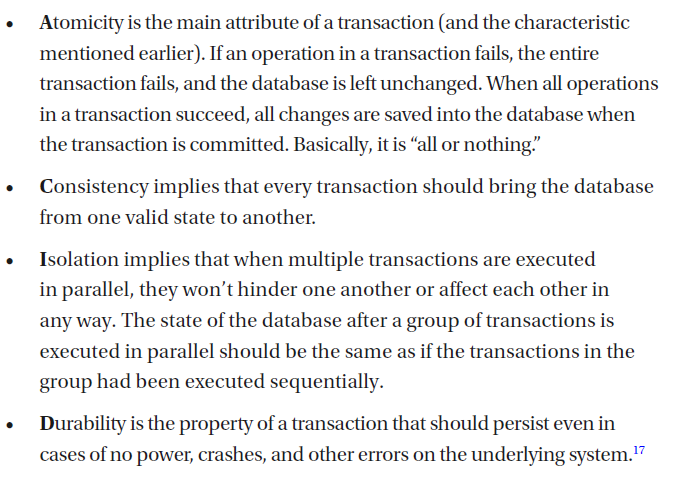
Types
Global transactions
Global transactions let you work with multiple transactional resources, typically relational databases and message queues. The application server manages global transactions through the JTA.
Local transactions
Local transactions are resource-specific, such as a transaction associated with a JDBC connection.
Declarative vs programmatic
The Spring Framework provides both declarative and programmatic transaction management. Most users prefer declarative transaction management, which we recommend in most cases.
With programmatic transaction management, developers work with the Spring Framework transaction abstraction, which can run over any underlying transaction infrastructure. With the preferred declarative model, developers typically write little or no code related to transaction management and, hence, do not depend on the Spring Framework transaction API or any other transaction API.
Spring Transaction management
A transaction strategy is defined by a TransactionManager
, specifically the org.springframework.transaction.PlatformTransactionManager
interface.
public interface PlatformTransactionManager extends TransactionManager {
TransactionStatus getTransaction(TransactionDefinition definition) throws TransactionException;
void commit(TransactionStatus status) throws TransactionException;
void rollback(TransactionStatus status) throws TransactionException;
}
Several implementations of PlatformTransactionManager are available: DataSourceTransactionManager, JmsTransactionManager, JpaTransactionManager, WebLogicJtaTransactionManager, WebSphereUowTransactionManager.
To enable Spring Transaction support:
- declare a PlatformTransactionManager bean.
- declare the transactional methods.
- add @EnableTransactionManagement to configuration class.
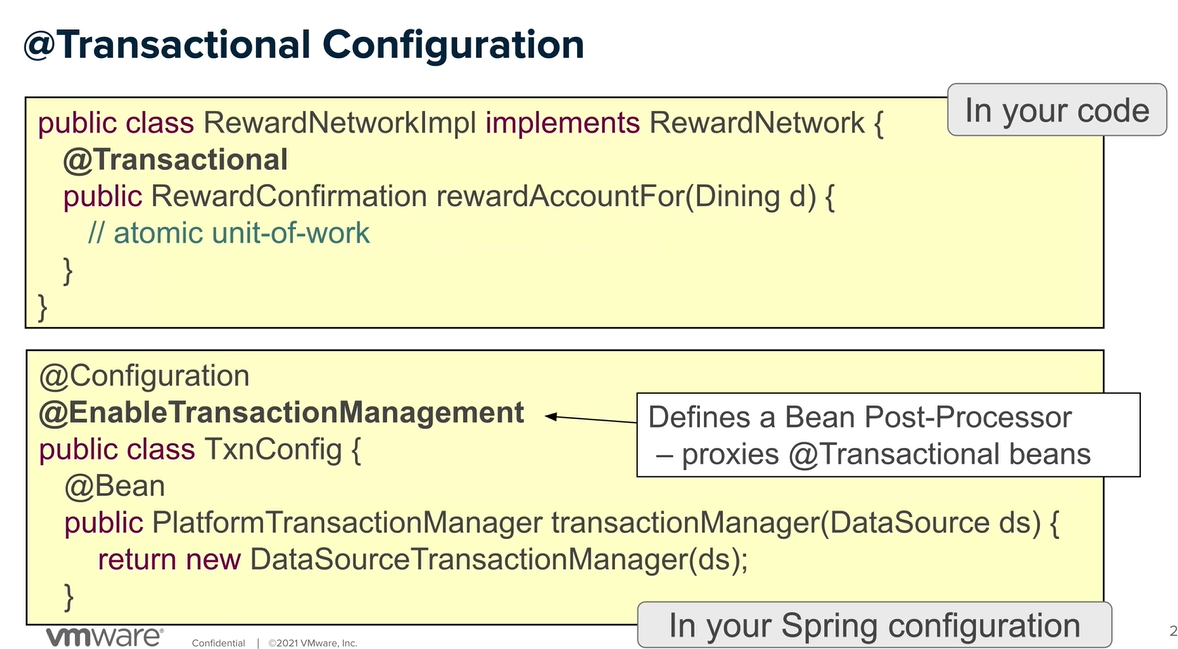
Declarative configuration
The Spring Framework’s declarative transaction management is made possible with Spring aspect oriented programming (AOP).
Roolback rules
In its default configuration, the Spring Framework’s transaction infrastructure code marks a
transaction for rollback only in the case of runtime and unchecked exceptions. Checked exceptions that are thrown from a transactional method do not result
in rollback in the default configuration.
Specific types can be marked for rollback using rollback rules. When using @Transactional, rollback rules may be configured via the rollbackFor / noRollbackFor and rollbackForClassName / noRollbackForClassName attributes.
When an exception type is specified as a class reference its fully qualified name will be used as the pattern. Consequently, @Transactional(rollbackFor = example.CustomException.class) is equivalent to @Transactional(rollbackForClassName = “example.CustomException”).
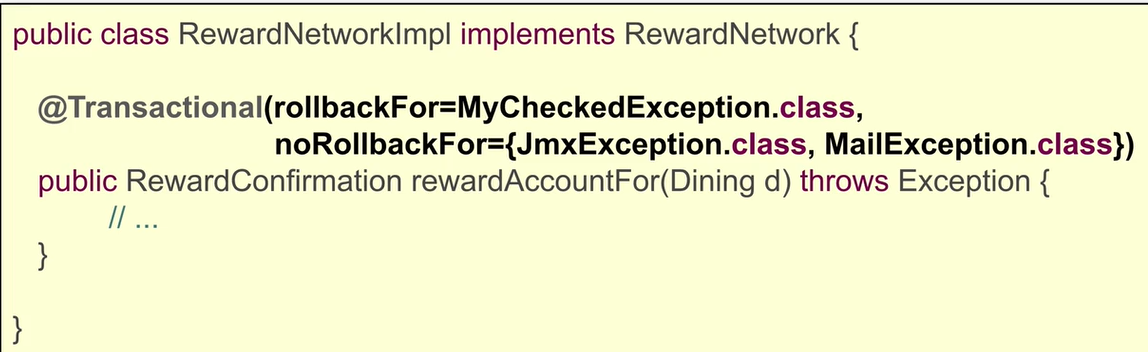
Transaction annotation
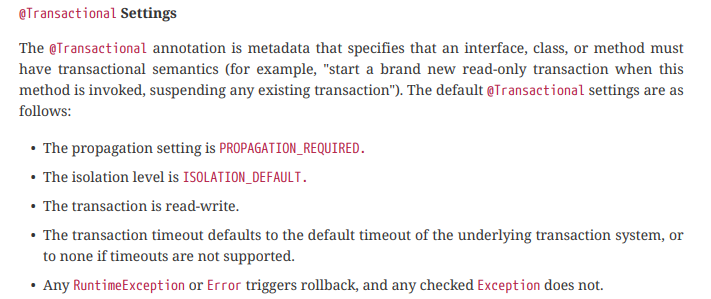
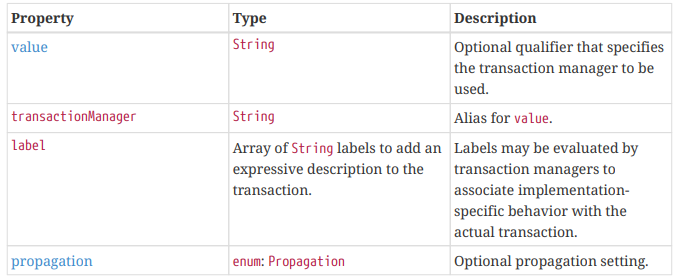
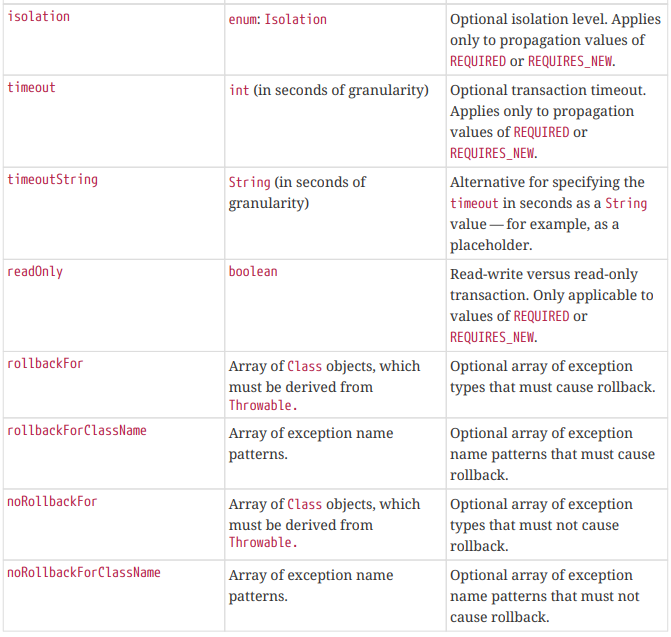
When you use transactional proxies with Spring’s standard configuration, you should apply the @Transactional annotation only to methods with public visibility. If you do annotate protected, private, or package-visible methods with the @Transactional annotation, no error is raised, but the annotated method does not exhibit the configured transactional settings.
The @Transactional annotation can be used at class level, making all the methods in class to became transactional, however, this configuration can be overridden by a @Transactional at method level.
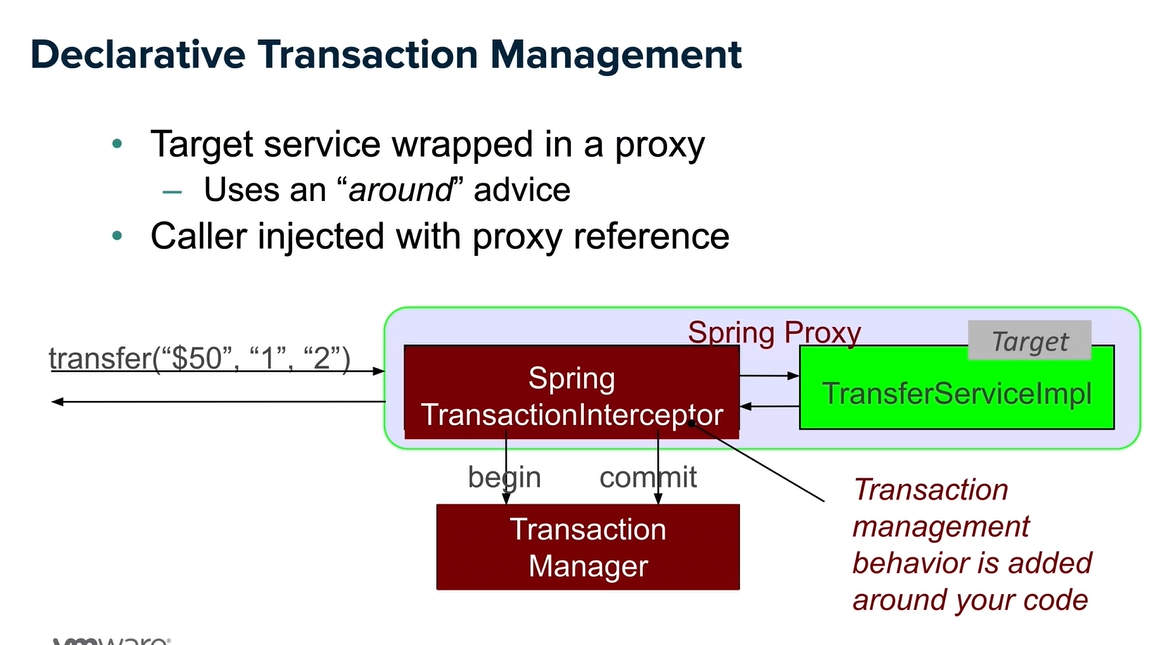
https://docs.spring.io/spring-framework/reference/data-access/transaction.html
Programmatic Transaction Management
The Spring Framework provides two means of programmatic transaction management, by using:
- The
TransactionTemplate
orTransactionalOperator
. - A
TransactionManager
implementation directly.
The Spring team generally recommends the TransactionTemplate
for programmatic transaction management in imperative flows and TransactionalOperator
for reactive code. The second approach is similar to using the JTA UserTransaction
API, although exception handling is less cumbersome.