PropertySource
Represents loaded properties from runtime environments.
A PropertySource is a simple abstraction over any source of key-value pairs, and Spring’s StandardEnvironment is configured with two PropertySource objects — one representing the set of JVM system properties (System.getProperties()) and one representing the set of system environment variables
(System.getenv()).
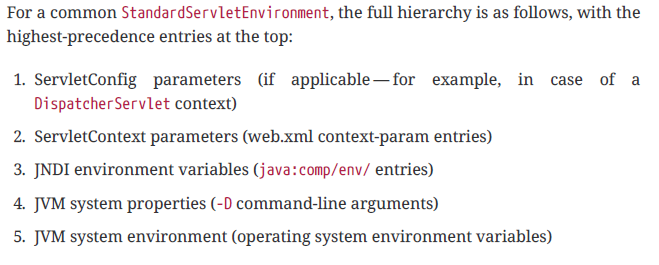
Profiles
Beans can be grouped in profiles, these can represent environment, implementation or deployment platforms.
@Profile({exp,exp1,…})
Create a new Profiles instance that checks for matches against the given profile expressions.
The returned instance will match if any one of the given profile expressions matches.
The following operators are supported in profile expressions.
!
– A logical NOT of the profile name or compound expression&
– A logical AND of the profile names or compound expressions|
– A logical OR of the profile names or compound expressions
Configuration:
@Configuration
@Profile("embedded")
public class DevConfig {...}
or
@Configuration
public class Config {
@Bean(name="datasource")
@Profile("embedded")
public Datasource getDataSourceDev(){
...
}
@Bean(name="datasource")
@Profile("!embedded")
public Datasource getDataSourceProd(){
...
}
}
Activate profile:
-Dspring.profiles.active=embedded,jpa
System.setProperty(“spring.profiles.active”,”embedded,jpa”)
Tests
Tests profile can be annotated with: @ActiveProfiles(“dev”)